This post is based on an unofficial guide. For best results, it is important that you are extremely familiar with Chapter 51. This post assumes that the you are aware of the structure of the pre-release material. Information beyond the video is tailor made for version 21.
Data Declaration
Data Type | Data Name | Explainer |
STRING[ ][ ] | bookingTable | A 2D array to store the name and car license. Each [ ] [ ] represents a slot on a particular day. |
INTEGER | today | A variable to store the current day within the static two week period. Must be a value between 1 and 14. |
INTEGER | customerSlot | A variable to store the slot that was booked. May be a value between 1 and 20. However, in the case that no slot is available the value will be -1. |
STRING | bookingReference | A variable that stores the name and car license to indicate a booking e.g. "Jaylan Waters, FGH 345". |
INTEGER[ ] | accessesibleStatCount | An array that keeps track of bookings of accessible slots on each day. The data stored is then used to calculate stats. |
INTEGER[ ] | generalStatCount | An array that keeps track of bookings of general slots on each day. The data stored is then used to calculate stats. |
Data Input Validation
The program prompts the user to enter the day in which the user requests a parking slot. Any data entry must be validated and in this case the day has to be greater than today and less than or equal to 14. This validation is called a range check. The mechanism of this kind of check is illustrated in the flowchart on the left. Note that there is a variable declaration for today which is a program variable that stores the current day within the static two-week period. The diagram explains that the user cannot request a parking slot in the past, on the current day and outside the static two-week period.
Data Structures
We are using a two-dimensional (2D) array to represent all parking spots that can be booked within a static two-week period. This data structure can be visualised as a simple grid with rows and columns. The bookingTable[ ][ ] has 20 rows, because there are 20 parking slots, and 14 columns because there are 14 days within the static two-week period. When there are no bookings the entire data structure is empty. However, the array gradually gets filled as customers successfully book parking spots.
We will demonstrate this concept through the following table. Most table cells are empty, however three cells are storing a bookingReference which is made up of the customer's name and a license registration code. The below indicates that a user booked the third parking slot on the first day, a different user booked the first parking slot on the second day, and, a user booked the second parking slot on the third day. Note that the fourth day remains fully available.
Slot/Day | Day 1 | Day 2 | Day 3 | Day 4 |
Slot 1 | | Paul Deguara, BHJ 345 | | |
Slot 2 | | | Erika Camilleri, FCB 123 | |
Slot 3 | Melinda Borg, XYZ 877 | | | |
When a customer wants to book a parking slot we need to locate the correct cell which is indicated by the parking slot and the day. Below is an example of a reservation of the twelfth parking slot on day six.
bookingTable[12][6] = "Customer Name, License Registration"
Expressing Algorithms
Hereunder is a flowchart that illustrates the logic behind Task 2. The program asks the user whether or not the parking slot needs to be accessible. When a user requests an accessible parking slot then a procedure nextAccessible() is called, which performs a linear search on bookingTable[ ][ ] for the first available parking spot on a particular day. The procedure returns a value between 1 and 20. When users opt for a general parking space, then a procedure nextGeneral() is called, which performs a linear search on bookingTable[ ][ ] for the last available parking spot on a particular day. The procedure returns a value between 20 and 6. Therefore, only users that need an accessible parking slot can book slots between 1 and 5.
Below we have provided pseudocode for the two different linear searches that take place.
day <-- 5
bookingTable[20][14] OF STRING
customerSlot <-- -1
// Linear Search Accessible
counter <-- 0
REPEAT WHILE counter < 20 AND customerSlot == -1
IF bookingTable[COUNTER][DAY] == NULL THEN
customerSlot = counter
END
COUNTER ++
END
// Linear Search General
counter <-- 20
REPEAT WHILE counter > 5 AND customerSlot == -1
IF bookingTable[COUNTER][DAY] == NULL THEN
customerSlot = counter
END
counter --
END
In both cases it is very possible customerSlot remains -1. When this happens, it indicates that there are no parking spots available for booking on the day requested and the user is presented with an error.
Data Analysis
Writing programs often makes it possible for us to perform data analysis. Data Analysis is the process of processing data in a way that helps us get useful information and insights about a situation. Task 3 is a menu-based data analytical program that is meant to be used by the people administering the car park. The process of data analysis involves a number of activities listed hereunder:
Data Modelling

The program does data processing within a static two-week period. This means that if a static two-week period started on the 10th of October 2022, then today is the Day 4 (as per calendar on the right). Day 14 is the 23rd of October and the last of the static two-week period. On the 24th of October the static two-week period rolls over so that it is not Day 15, but Day 1. To keep track of the type of parking spaces booked we have two integer arrays: accessibleStatCount[ ] and the generalStatCount[ ]. Every index in the array corresponds to a day between 1 and 14. The small pseudocode snippet below illustrates this concept.
accessibleStatCount[14] OF INTEGER
generalStatCount[14] OF INTEGER
accessibleBookingsDay4 = accessibleStatCount[4]
generalBookingsDay4 = generalStatCount[4]
Collecting Now that the models are in place, data collection can occur whilst the users are making their bookings. The following flowchart explains that the data collection happens right after the booking reference is saved into the bookingTable[ ][ ] which is referred to as saveBooking() to keep the diagram clean and easy to read.

Perform Analytical Functions A menu-driven program is available for a user who needs to see the data collected in a certain format. The menu displays analytical options to process raw data that was collected.
The user is prompted to enter an option from "A" to "F". This user input is validated to make sure that the option exists, this is called a constraint check. When the user gives a valid option, we can use a conditional statement like a switch statement in Java to invoke the appropriate function.
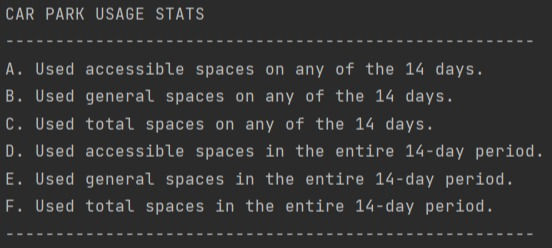
In this aspect of the program there aren't any novel techniques to talk about that were not described before. The most complicated option is "F" and below is a pseudocode snippet that can give you a clear idea of what the analytical functions entail. We declared a variable total and initialised it to zero. Then, we loop through indices 1 and 14 and perform a recurring total. The final result is the number of spaces booked in total within the entire static period regardless of whether it was accessible or general.
TOTAL <-- 0
FOR INDEX FROM 1 TO 14
TOTAL = TOTAL + GENERAL_STAT_COUNT[INDEX] +
ACCESSIBLE_STAT_COUNT[INDEX]
END
OUTPUT "Used total spaces in the entire 14-day period: " , TOTAL
Interpret Results
This is really up to the user doing the analysis and the program does not do interpretation of results.
Cleaning
When a static two-week period rolls over all of the data needs to be cleaned out, including the data models that are used for analytics. The data structures that require cleaning are: bookingTable[ ][ ], accessibleStatCount[ ] and generalStatCount[ ]. In this case, cleaning is just deleting the data that was already there. Deleting data in an array is quite simple.
// Deleting bookings data
FOR slot FROM 1 to 20
FOR day FROM 1 to 14
bookingTable[slot][day] = NULL
END FOR
END FOR
// Deleting stat data
FOR index FROM 1 to 14
accessibleStatCount[index] = 0
generalStatCount[index] = 0
END
Where can I see some code please?
Follow this link to see a solution in Java.
Comments