In the last lesson we set up Maker Uno X and connected it to our computer. We did not have much control over the code execution during run-time. In this post we will execute the code on demand and learn about simple electric circuits.
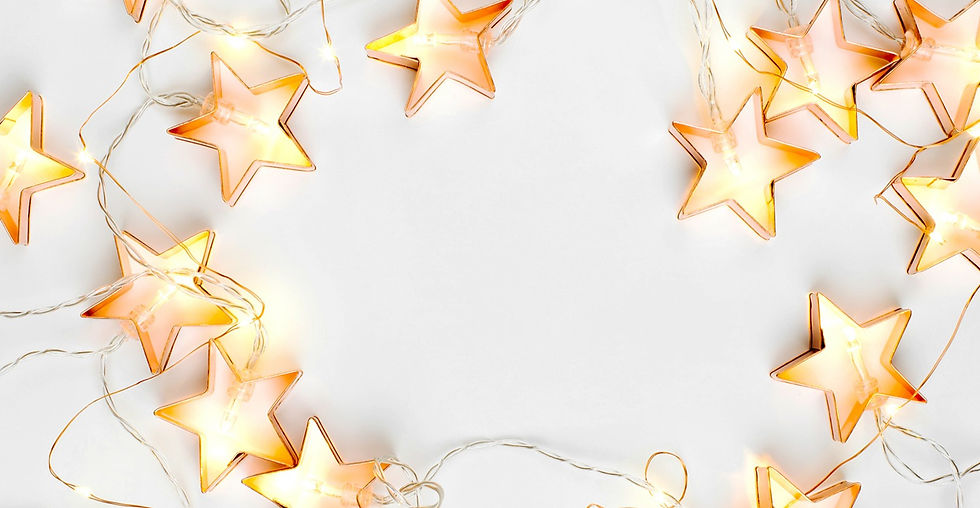
Glossary
GND | A flow of charge. |
resistor | A complete circular path for electricity to flow. |
buzzer | An electrical device that makes a buzzing noise and is generally used for signalling. |
diode | A two-terminal electronic component that has low resistance in one direction, and high resistance in the other. |
Many people asked me what I teach in Computer Science because it is so vast and multidisciplinary. Before I answer their question I always ask what they think it is first, mostly to buy me time to think about my answer. Unsurprisingly there are many points of view.
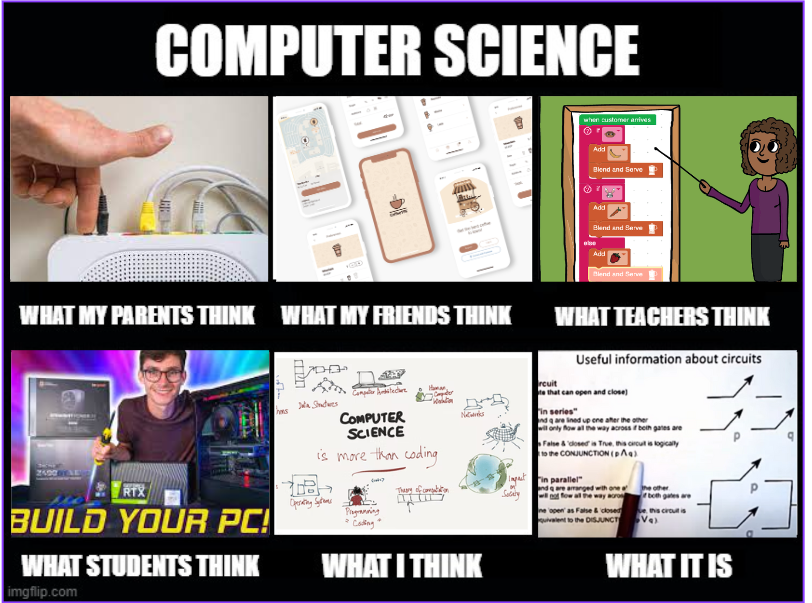
Even though for me Computer Science means changing the world by thinking and creating, there is in fact a more accurate but less heroic definition.
In these sessions with our Maker Uno X we will be stripping out the many layers of abstraction that we take for granted. And, we will see that...
computer science is the study of how one can control the flow of electricity in order to simulate logical thinking.
The way electricity flows in our hardware is the secret sauce. We do not work with electricity directly of course, but the code we write in the Arduino IDE is a representation of how electricity should flow in our board. We will also design additional physical pathways in order to achieve more interesting results.
First things first, the simple circuit
This video provides a very good introduction to electric circuits. Watch it now 👇.
Hereunder we list the key takeaways:
There is a law that states energy cannot be created or destroyed, energy is however converted from one form to another. This implies that any circuit we build needs an energy source. In the above explanation, the energy source is a battery. We, on the other hand, connect Maker Uno X to the computer, and it acts as the power source.
The movement of particles that flows through the physical pathways in the circuit forms an electric current, enabling our output components to respond.
The absence of an electric current can only mean that the circuit is not closed! This can be intentional of course, e.g., through a switch. However, if we design a circuit and nothing happens when we toggle the switch on, then the circuit is not closed and somewhere a long the way we made a mistake.
In this lesson... 📝
A simple circuit with a flashing light from the Arduino Learning Guide.
Write a program to control an LED using the on-board push button toggle switch.
Constructing circuits using the breadboard
So far we've only been using onboard LEDs on the Maker UNO X board itself, but this is only to facilitate learning. Arduino projects require use of a breadboard, jumper wires and other components hooked up using circuit diagrams. In this lesson, we will get our hands dirty with some simple hooking up. We will learn more about the breadboard and some components available in the next lesson.
Here is a sample of a circuit diagram that we will be using in this lesson. It is the same type of diagram you will encounter in your coursework. Don't be intimidated by the wiring. You will always be provided with a circuit diagram to follow.
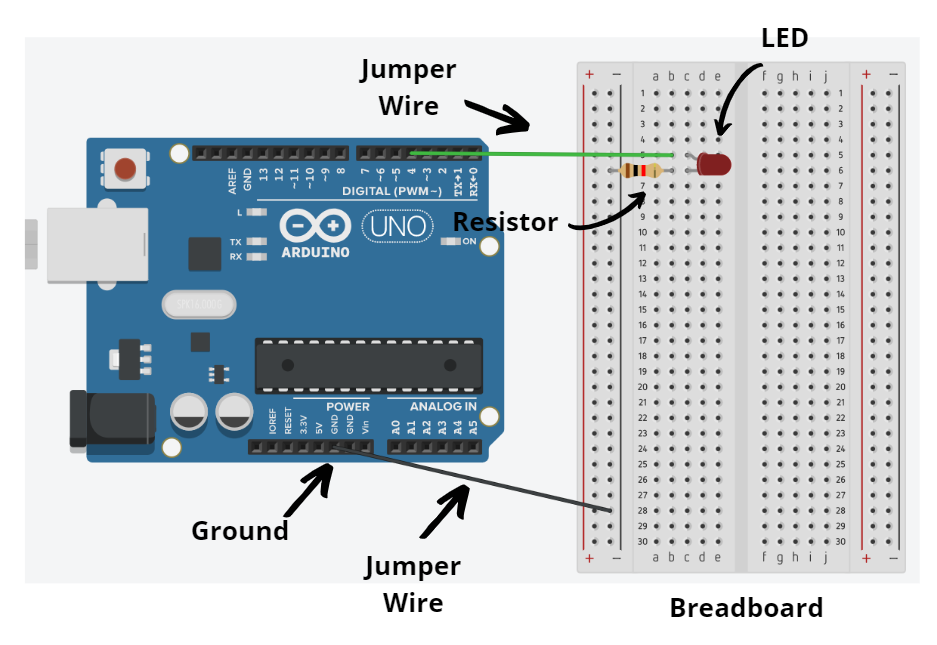
Breaking it down:
There is a black jumper wire from the ground on the board (GND) to the vertical (-) bus on the breadboard.
There is 1 LED
The LED has the short end along a horizontal bus with a resistor, and the long end on a horizontal bus with a green jumper wire. We use resistors to dim the LED output.
The green jumper wire connects to pin 4 on the board.
You can see how the circuit is closed in this case by following the yellow dashed lines in the following diagram:
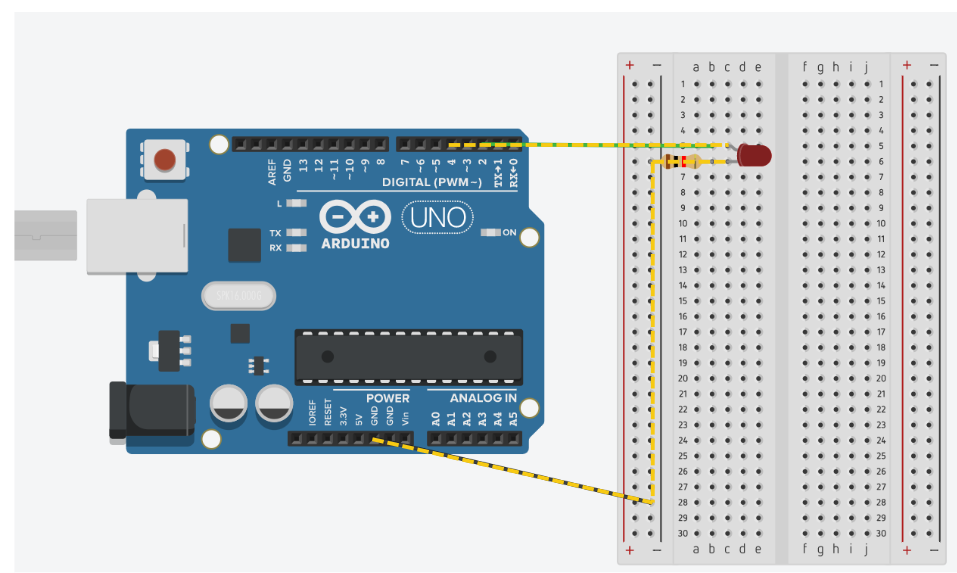
Have a go at constructing this circuit on your Maker UNO X, paying careful attention to the positioning of each element. Then run the following code:
void setup() {
pinMode(4, OUTPUT);
}
void loop() {
digitalWrite(4, HIGH);
delay(300);
digitalWrite(4, LOW);
delay(300);
}
Your LED should keep flashing at 300 ms intervals. Notice how we have removed the exit() line to allow Arduino to keep looping.
Using the on-board push button as a switch
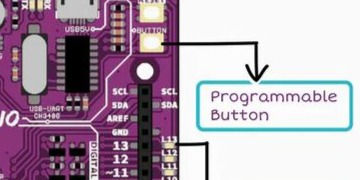
The Maker Uno X has a programmable button right underneath the reset button. We can write code in the Arduino IDE that will only execute when the button is pressed. As an example, we can treat the button like a toggle switch for one of the LEDs on the board. The LED will only light up whilst the button is pressed, otherwise, the light will be off.
We can update our previous Arduino code for this simple push button light is as follows:
void setup() {
// this is where we setup our board
pinMode(2, INPUT_PULLUP); // onboard push button
pinMode(4, OUTPUT); // using pin 4 as output
}
void loop() {
// if the input is pressed, light up output 4
if ( digitalRead(2) == LOW ) {
digitalWrite(4, HIGH);
} else {
digitalWrite(4, LOW);
}
}
Breaking it down
We first need to let Arduino know that we are expecting an INPUT signal by adding this line in the setup block:
pinMode(2, INPUT_PULLUP); // onboard push button
On the Maker UNO X, this refers to the onboard push button.
Next, in the loop block, we need to tell Arduino to do one of two things (LED on or off) based on whether the pushbutton is pressed or not.
Let's visualise this concept by drawing a simple flowchart.
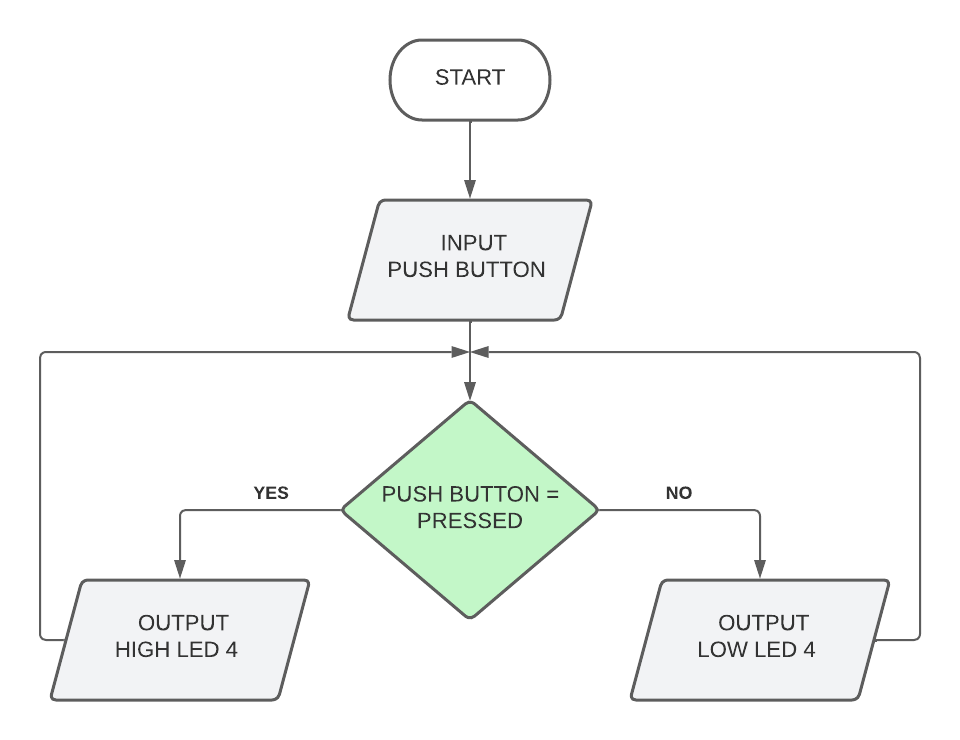
You should notice that we included a conditional statement. A kind reminder to refer to Chapter 51 if you cannot remember what this is. In programming languages, we normally refer to this kind of branching as an if-else statement. C++, the language we are using in Arduino, is no exception:
/* if we are pressing the on-board push button */
if ( digitalRead(2) == LOW ) {
digitalWrite(4, HIGH);
} else {
digitalWrite(4, LOW);
}
In our case, the if-condition will be a digitalRead, since we are reading an input.
You might be thinking that this feels like cheating... we did not really build a switch. You are right, but we are not too far off either! Recall that the electrical current is the phenomenon that powers up our output components. When we are pressing the button, we are creating a resistance. This does not create a break in the circuit, as a switch would do, but it will make it very difficult for the particles to pass through. In fact, we make it so difficult that the electrons give up and find another way to move around. We might get a few outliers, but not enough to power up the LED.
The output components on the board are set up in a parallel circuit, meaning that all the electric components do not share one circuit loop. Each output component is in its own circuit loop. When we create a resistance, we are only affecting one of the loops, so electrons are happy to flow through other available pathways. Think of it as us putting a really tiny no entry sign ⛔ for the electrons.
IF Statement and Logical Expressions
We saw earlier, that the if-else statement in C++ is:
if ( /* logical expression */ ) {
/* code that runs when true */
} else {
/* code that runs when false */
}
The if-else statement evaluates the logical expression inside the round brackets "(...)".
When logical expression is evaluated to "true", code inside the if block is run.
When the logical expression is evaluated to "false", code inside the else block is run.
We normally construct logical expressions to check whether something has a particular value. For example, at any point in the program, a digitalRead of a pin can gives us a value of either LOW or HIGH. We would want to do something in particular only when digitalRead is LOW so we use the equality operator (==) like so:
if ( digitalRead(2) == LOW ) {
/* code that runs when digitalRead(2) has a LOW value */
}
Other Comparitive Operators for Logical Expressions
Operator | Description | Notes |
!= | Not Equal | -- |
>, >= | Greater Than, Greater Than or Equal | Compare numbers |
<, <= | Less Than, Less Than or Equal | Compare numbers |
Challenge: Retro Christmas Lights 🎄
Try to enhance your Retro Christmas Lights project so that you make use of:
LEDs on the breadboard;
Resistors;
And, the onboard pushbutton.
Here's a sample of what you should end up with:
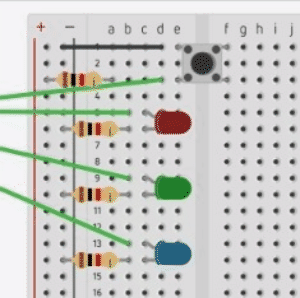
Feeling adventurous? Add some sound!
Maker Uno X has an on-board buzzer that can output sound. It should be easy enough to make the LEDs flash to a simple tune by referencing the official Arduino Learning Guide on page 47.
In the setup section:
pinMode(8, OUTPUT); // buzzer
In the loop section:
tone(8, 330, 200); // play E for 200ms
where the first parameter is the pin, the second is the tone frequency, and the third is the duration in ms.
Try enhancing your Christmas lights further to add some sound to the blinking!
Comments