⏰ 45 minutes — 1 hour 💪 Moderate Effort
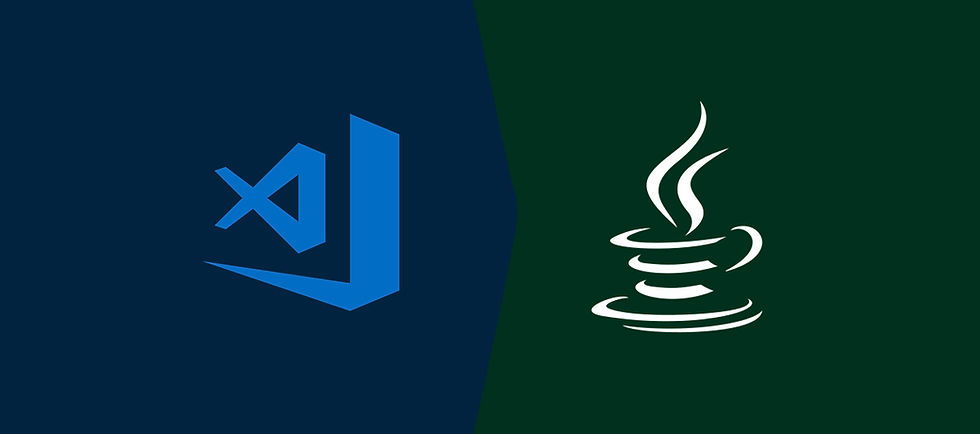
This post is intended for students who are already introduced to Java (or are familiar with a similar language) and would like to level up their programming experience. Visual Studio Code is a great first IDE. It has the feeling of a lightweight text editor but packs in just enough power to make you feel like a pro!
Install The Coding Pack For Java
We need to install Visual Studio Code. Since Java is such a popular language you can immediately install a coding pack from here (thank you Microsoft!). As stated in the docs the coding pack will include VSCode, Java Development Kit and Java Essentials like IntelliSense and Auto Build Tools 🎊. If you are already using VSCode you can just install the extension pack!
Meet Your IDE
This video walks you through the UI and provides an overview of the basic features.
A Quick Peek Under The Hood
We are just going to make sure that everything is as we expect under the hood to be able to develop, compile and run Java programs on our computer.
Time for a flashback...
When starting out you were probably told that Java is very popular because it is platform-independent. This is true because any computer that has a Java Runtime Environment (JRE) installed can run Java applications. The JRE is a thin layer on top of the Operating System which is composed of Java specific dependencies and a Java Virtual Machine (JVM). The JVM is the special container that is responsible for running Java applications. It has many important capabilities, for example asking the OS to allocate memory for objects and letting the OS know that some memory is freed up when the application closes. It is not possible to run Java applications outside of a JVM.
Us developers obviously need a JVM but we need more than that — like a compiler — which is why we install the Java Development Kit (JDK). When we code our programs in Java we do not really have a particular machine in mind and the compiler does not really care either. When projects are built for distribution the end-result is Java byte code. On the other hand, JREs are environment specific so it is within the JVM that the byte code is converted to pure machine code. For us developers this is great news because we do not need to move mountains to find multiple compilers (Objective-C I am looking at you). We can just build one version of the program. Yay! Back to VSCode to find our JDK installation...
Go to Manage > Command Palette... ( View > Command Palette... on 🪟🪟) and in the combo box on focus search for "Configure Java Runtime". The first item in the list should be the correct one. When you click on it a new tab should appear and under "Installed JDKs" you should see at least one entry. Fantastic!
"But I do not see a JDK installed..." If you just installed the extension pack earlier on it does not include a JDK. Not to worry though because you can fix this by downloading it from this screen.
If the installation worked perfectly, when you open a new Terminal (or PowerShell in Windows) and type in java you should see the command help menu. This means that the tools provided by the JDK can be accessed from anywhere and not just within VSCode.
Hello, World!
Let's just do a routine check to make sure everything is working.
In VSCode go to Manage > Command Palette... and in the combo box on focus search for "Create Java Project". Again, the first item in the list should be the correct one. When you click on it, the combo box changes and offers multiple options. Select the option "No build tools". We have just created a typical 'Hello, world' program (without writing a single line of code ourselves) so right off the bat you should be able to Run ▶️ it. Pressing Run Java simply executes the command...
/* Run App.jar */
java src/App.java
I just want to remind you that runnable java files must implement the main method...
public static void main(String[] args) throws Exception {
/* Your code goes here */
}
You are now set up to design and test programs on your computer using Java. In order to write programs and run them only on your computer you do not need to continue following this post. However if you would like to close the loop we are going to compile our program and build the jar file in the next section.
Compile And Build The JAR File
After all that hard work coding 😛 it is time to compile our code and build it for distribution.
Earlier in this post we mentioned that the JDK includes all the development tools required. A lot of the tools are small utility programs written in Java. You should be able to find them on your computer. They are typically kept in a bin (short for binary) folder inside the JDK installation. Have a look at some of the files... one of them is named java — this is the tool we just used to run the 'Hello, World' program in VSCode.
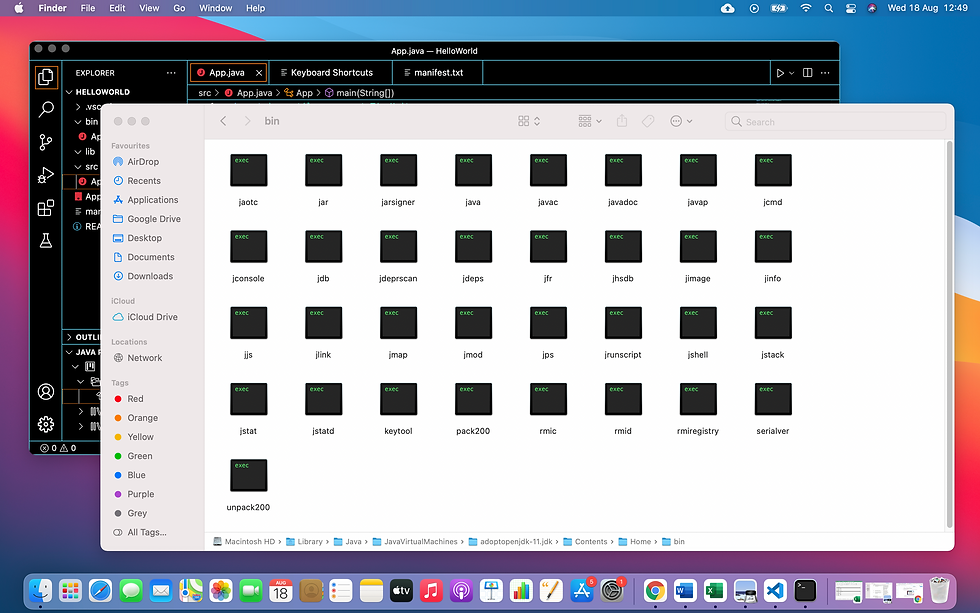
Compiling
Go to the terminal inside VSCode and use the javac tool to compile all your source code, in other words, all files that contain the .java extension. You may simply copy the command below into the terminal window. The small words that start with a hyphen are command options. Apart from telling the compiler to look for any source code under the src folder, we are also passing in the class path [-cp] and the destination [-d] folder for our java bytecode.
/* Compile */
javac -cp src src/*.java -d bin
All we need to do now is nicely package the java bytecode in a single executable JAR file. This is the kind of file that you can give to your friend for instance to get an opinion about an application your are building.
Building the JAR
Let's create a manifest.txt file so that our application can be tagged with a version. Download a copy and place it inside the root folder of your project. Use the command below to build the JAR file. In the command we are passing in the desired name for the executable, the location of the manifest file, the location of the folder that contains our java bytecode and where the executable should be created (a dot indicates the current location).
/* Build Jar File */
jar -cvfm App.jar manifest.txt -C bin .
Execute the JAR File
You should be able to run App.jar now by using the command...
/* Run JAR File */
java -jar App.jar
Why go all through this trouble? You can test the application on other machines easily and share your program with friends without having to give up the source code. To test the idea simply:
Copy an instance of App.jar onto the Desktop
Open a fresh terminal window outside of VSCode
Navigate to the Desktop folder
Run App.jar using the same command
Happy coding!
Comments