In this last part of our CPU section, we explore how assembly language is used to make it easier to program, instead of using machine code.
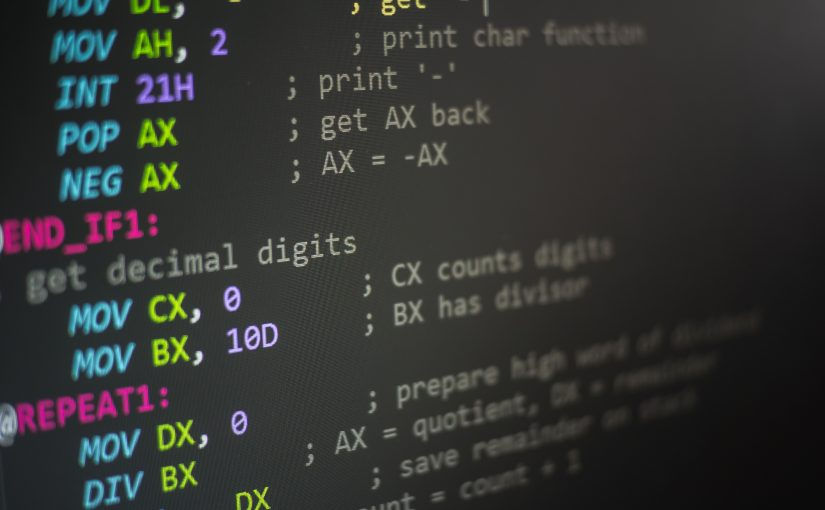
Glossary
Machine Code | Language consisting of binary or hexadecimal instructions. |
Assembly Language | A low-level programming language designed for a specific type of processor. |
CPU Instruction Set | A group of commands for a central processing unit (CPU) in machine language. |
Assembler | A program that takes basic computer instructions and converts them into machine code. |
Opcode | The portion of a machine language instruction that specifies the operation to be performed. |
Operand | The value on which top implement the instruction e.g. 5. |
Mnemonic | A short-code for a machine instruction e.g. LDA. |
Machine Code vs Assembly Language
In Chapter 31, we'll talk about different levels of languages on this spectrum, including high-level languages. In this chapter, we're going to focus on low-level languages.
Machine code is the lowest level language, closest to the hardware, working with 1's and 0's. Assembly is also a low-level language, but it is slightly easier to work with than machine code because it uses mnemonics, which are short-codes that are used to help the programmer remember what it means. An assembler takes a program written in assembly and translates it to machine code.
A CPU instruction set is a group of instructions that are supported by the CPU. This will vary based on the CPU vendor, so a particular assembler is required for each CPU instruction set.
A Sample Assembly Program
Here is an assembly program with 4 lines:
To explain:
We're not using 1's and 0's. Opcodes like LDA are mnemonics to help the programmer remember the instruction code for a particular function.
Operands are the pieces of data the function needs to work with.
The program is instruction focused meaning that it tells the computer step by step what to do.
Understanding Assembly Instructions
Here are some more assembly instructions you can probably understand. With these, we can build basic programs that have variables, constants, conditions and loops.
Example 1: The IF Statement
Here is a basic IF statement in Python:
if var1 == var2:
x = 1
else:
x = 2
And here is what it would look like in assembly:
1 MOV eax, var1
2 CMP eax, var2
3 JNE elsepart
4 MOV X, 1
5 JMP next
6 elsepart: MOV X, 2
7 next: HLT
Example 2: A Looping Statement
Have a look at this Python code:
a = c
a = a * 2
while a != 0:
a = a - 1
And the equivalent assembly code:
1 LDA C ;Load contents of C into accumulator A
2 MUL #2 ;Multiple accumulator by 2
3 lab1: SUB #1 ;Subtract contents of accumulator by 1
4 JNZ lab1 ;If not zero, jump to label lab1
5 HLT ;Stop the program
Fetch Decode Execute Cycle For An Instruction
In Chapter 26 we have outlined the Fetch Decode Execute Cycle.
The clever animation on the left illustrates how an instruction gets processed by the CPU.
Let us break down the steps for the first instruction in the video:
LOAD 5 # Loads the value in memory location 5 in the CPU registers
CU fetches the instruction from memory.
CU decodes the instruction by fetching the value in memory location 5 and translating the opcode LOAD into signals.
CU signals automatically execute this particular instruction.
The result is that the value is stored into a special-purpose register called the Accumulator.
Comments